WooCommerce plugin development allows you to create custom solutions to enhance the functionality of your online store.
This guide covers everything from setting up your development environment to coding best practices and ensuring your WooCommerce plugin is secure, efficient, and tailored to your needs.
In this tutorial, we will explore the development of WooCommerce plugins, how to develop your plugin, and what to keep in mind during this process.
First up are tools that may come in handy, best practices for doing it right, and a few bonus tips to ensure your WooCommerce plugin is top-notch.
Table of Contents
What is a WooCommerce Plugin?
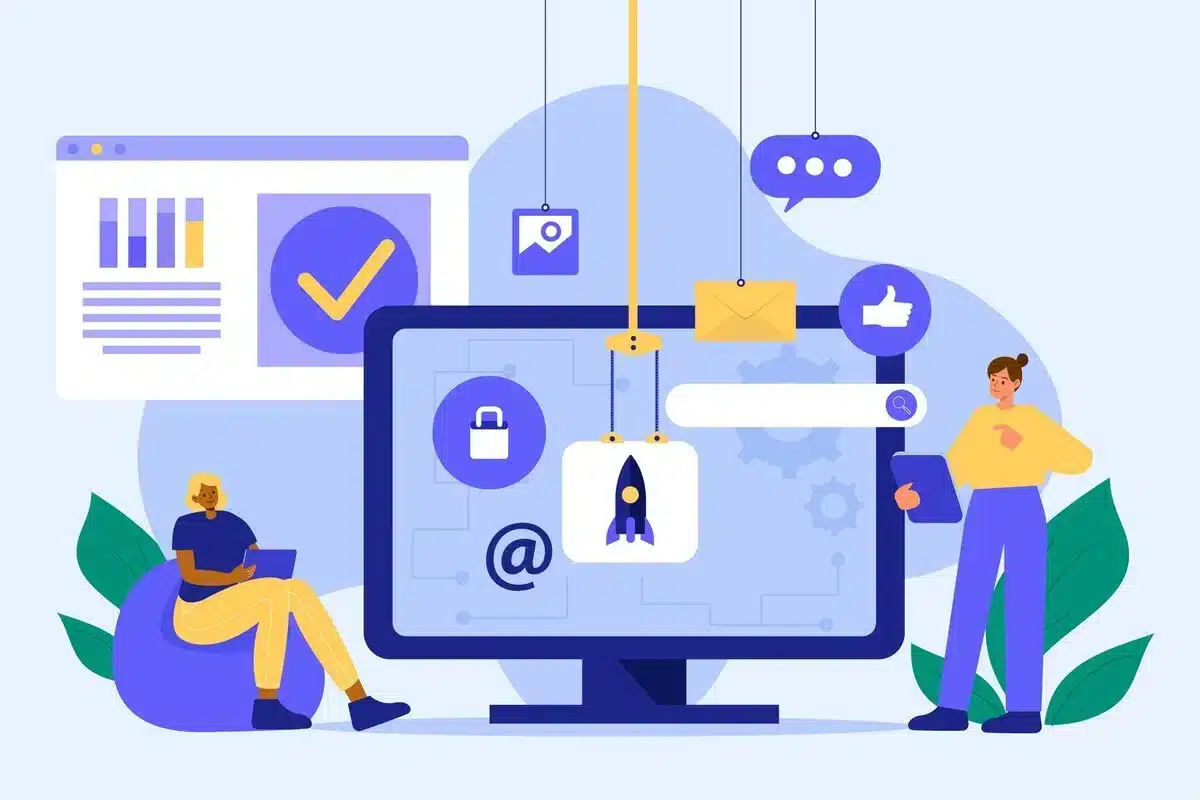
Before we develop the plugin, let’s define it. A WooCommerce plugin is an add-on software that extends and enhances the core functionality of WooCommerce, which is itself a plugin to the WordPress core.
WooCommerce plugins allow you to add, modify, or extend the capabilities of your WooCommerce store. For instance, with WooCommerce plugin development, you can:
- Add new payment gateways.
- Integrate third-party services like CRMs or marketing tools.
- Create custom shipping methods.
- Personalize the checkout experience.
- Implement advanced analytics or reporting.
The beauty of WooCommerce plugin development lies in its flexibility. If WooCommerce’s default features aren’t sufficient for your business, this can bridge that gap with a customized solution.
Why WooCommerce Plugin Development is needed
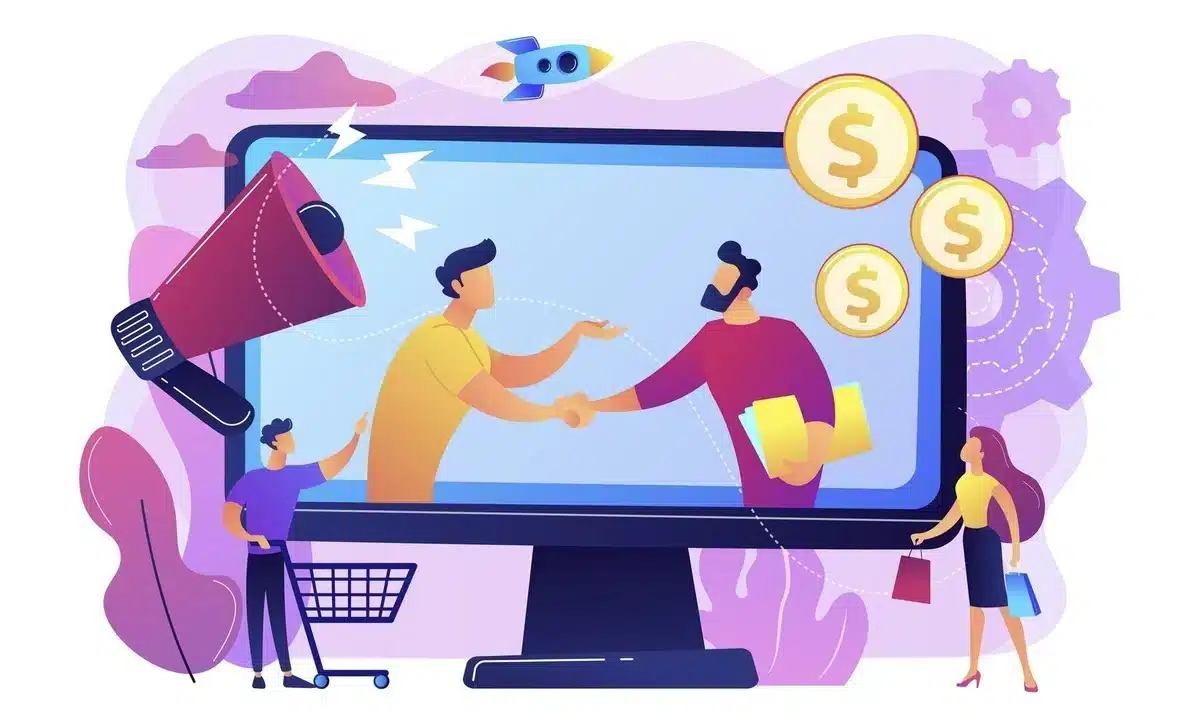
WooCommerce plugins come in handy when:
- Unique Functionality: If your store has special requirements that existing plugins can’t handle, then WooCommerce plugin development is the perfect solution.
- Full Control: Custom plugins give you complete control over your store’s features, behaviour, and performance.
- No usable plugin in view: If you have a set of requirements that no plugin can fulfil, your best option is to develop it by hand.
- Integration with other third-party services: The plugins support the integration with third-party services, such as CRM or marketing tools, to enhance the store’s capabilities.
- Versatility with no limits: From minor adjustability adjustments to complex integration constructions, using WooCommerce plugins gives you flexibility and possible growth without limits.
Whether you’re adding a simple tweak or developing a complex integration, WooCommerce plugins provide endless possibilities.
Prerequisites for WooCommerce Plugin Development
Before you start writing code, here are a few things you need to have in place:
- Knowledge of Basic PHP: Plugins for WooCommerce are written in PHP, so we need to have a decent understanding of it.
- Knowledge of WordPress Hooks: Hooks (actions and filters) are essential for WooCommerce plugin development. They allow you to modify core functionality without altering the base code.
- Familiarity with WooCommerce: Knowing how to navigate WooCommerce will help you determine where to put your new functionality.
- Ensure HPOS Compatibility: WooCommerce’s High-Performance Order Storage is a newer system that improves performance. Developers should ensure their plugins are compatible with HPOS for better future scalability.
- Local Development Environment: Set up a local environment to test your plugin. Tools like Local by Flywheel, XAMPP, or MAMP may be very helpful in this process.
- Text Editor or IDE: For your development work, you will require a code editor like VS Code, Sublime Text, or PHPStorm.
Step-by-Step Guide to WooCommerce Plugin Development
1. Plan Your Plugin
- Define the functionality: What does your plugin need to do? Write down the main features and the problem it solves.
- Research: Check for existing plugins that do something similar. You can draw inspiration from them or see how they solve certain problems.
- Set clear goals: Make sure you know what you want your plugin to achieve.
2. Set Up the Plugin Folder
- Navigate to your
wp-content/plugins/
folder in your WordPress installation. - Create a new folder for your plugin. It’s good practice to name the folder after your plugin, like
my-awesome-woocommerce-plugin.
3. Create the Main Plugin File
- Inside your plugin folder, create a PHP file named after your plugin, like
my-awesome-woocommerce-plugin.php.
- At the top of this file, add the plugin header
<?php /* Plugin Name: My Awesome WooCommerce Plugin Plugin URI: http://yourwebsite.com Description: A brief description of what your plugin does. Version: 1.0 Author: Your Name Author URI: http://yourwebsite.com Text Domain: my-awesome-woocommerce-plugin */
WordPress requires this header to recognize your plugin.
4. Initialize the Plugin
The next step is to set up a function that initializes your plugin. We can do this with the following code:
function mawcp_init() { // Your initialization code here } add_action( 'plugins_loaded', 'mawcp_init' );
This ensures your plugin’s code only runs after all the other plugins have loaded.
5. Add WooCommerce Compatibility
WooCommerce is not always activated, so we need to check if WooCommerce is running before executing your plugin code:
if ( ! class_exists( 'WooCommerce' ) ) { return; }
6. Create the Core Functionality
The next step is to write the core logic for your plugin. Whether it’s adding a payment gateway, customizing checkout fields, or creating new product types, this is where we dive into coding.
For example, let’s say you want to add a custom fee to the WooCommerce cart:
add_action( 'woocommerce_cart_calculate_fees', 'add_custom_surcharge' ); function add_custom_surcharge() { global $woocommerce; $surcharge = 5.00; // Flat surcharge $woocommerce->cart->add_fee( 'Custom Surcharge', $surcharge ); }
7. Test Your Plugin
- Testing is an important step in plugin development. Test our plugin on a local or staging environment before deploying it to your live site.
- Make sure to test for various scenarios such as:
- Plugin conflicts.
- WooCommerce updates.
- PHP errors.
8. Include Admin Settings (Optional)
If your plugin needs admin settings, we can create a settings page in the WooCommerce admin menu. For example:
add_action( 'admin_menu', 'mawcp_create_menu' ); function mawcp_create_menu() { add_menu_page( 'My WooCommerce Plugin Settings', 'WooCommerce Plugin', 'manage_options', 'mawcp-settings', 'mawcp_settings_page', '', 110 ); } function mawcp_settings_page() { echo '<h1>WooCommerce Plugin Settings</h1>'; // Add form and settings fields here }
9. Documentation and Updates
- It is important to document your code and provide proper instructions for users to install and use your plugin. Good documentation will reduce the number of support requests.
- Stay updated with the latest WooCommerce and WordPress changes to ensure your plugin remains compatible.
Best Practices for WooCommerce Plugin Development
- Follow WordPress Coding Standards: Stick to the WordPress coding standards for better readability and compatibility.
- Use Hooks and Filters: Avoid directly hard-coding modifications in WooCommerce’s code; instead, rely on hooks and filters. This will make your plugin even more flexible and less susceptible to breakage when there are updates.
- Security Matters Sanitize: User inputs and escape outputs to prevent SQL injection or XSS.
- Keep it Light: Avoid bloating your plugin by not overdoing the code. Instead, focus on solving a particular problem efficiently.
- Make it Translation Ready: Use WordPress’s internationalization functions to prepare your plugin for translation.
Tools and Resources for WooCommerce Plugin Development
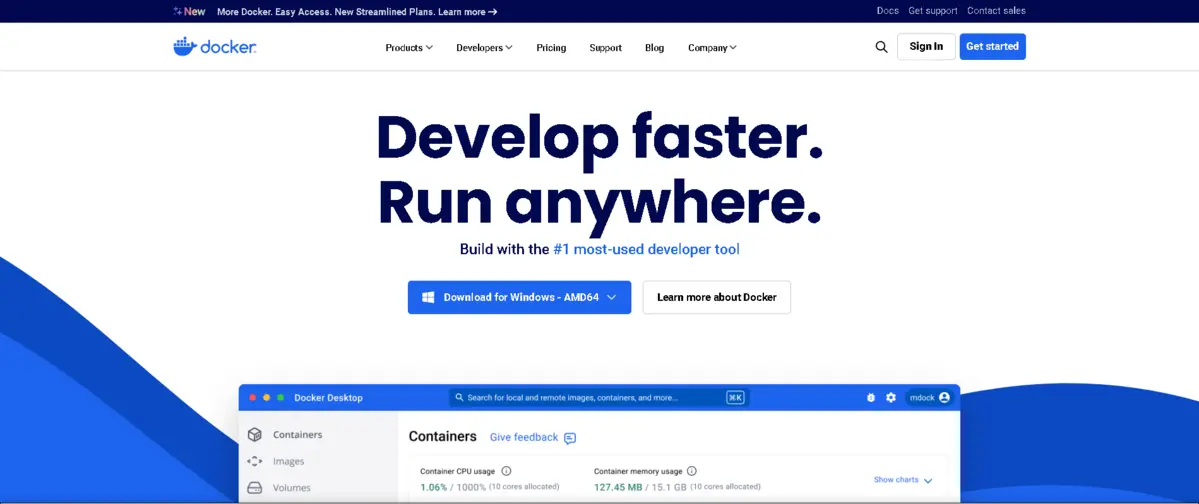
Here are some tools and resources that will help you in the development process:
- WooCommerce Documentation: The official WooCommerce documentation is a goldmine for developers. It covers everything from hooks and functions to REST API documentation.
- Local Development Tools: Tools like Local by Flywheel, XAMPP, or Docker will make your local development smoother.
- Code Editor: Choose a good editor or IDE. VS Code and PHPStorm are popular choices among WordPress developers.
- Git Version Control: Use Git for version control to track changes and collaborate with other developers easily.
- WP-CLI: The WordPress Command Line Interface (WP-CLI) can automate tasks like plugin activation or database updates.
Conclusion
While creating a WooCommerce plugin is daunting enough, once the basics are implemented, the journey begins to be exciting.
Well, if anything is possible, create a custom feature that you and your clients need to improve the functionality of your store or even create something unique for your clients. It’s possible with WooCommerce.
Now it’s your turn to jump into WooCommerce plugin development action. Start small. You’ll solve a very specific problem, and as you grow in confidence, you’ll create pretty sophisticated plugins that transform your WooCommerce into exactly what your business needs.
FAQs
What is WooCommerce plugin development?
WooCommerce plugin development is creating custom plugins to enhance or extend the functionality of WooCommerce, a popular eCommerce platform for WordPress.
Why should I develop a custom WooCommerce plugin?
Custom WooCommerce plugins allow you to add specific features tailored to your business needs, such as integrating third-party services, custom payment gateways, or unique product features.
Do I need coding skills for WooCommerce plugin development?
Yes, a basic understanding of PHP, WordPress hooks, and WooCommerce structure is required for successful WooCommerce plugin development.
Can I modify existing WooCommerce plugins?
Yes, you can customize or extend existing WooCommerce plugins through WooCommerce plugin development to fit your needs.
Is WooCommerce plugin development secure?
As long as you follow WordPress coding standards, sanitize inputs, and escape outputs, WooCommerce plugin development can be done securely.